Using the DNN Text Suggest Control
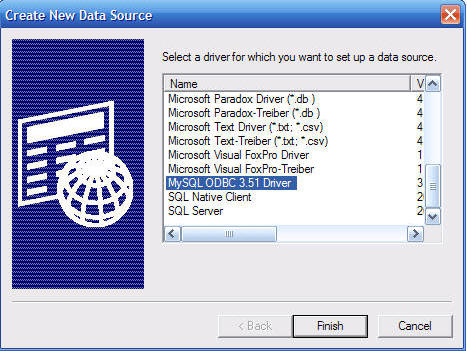
The DNNTextSuggest control is actually a very complex control. You can
go to the example website at:
http://webcontrols.dotnetnuke.com where you can test out all the DNN web
controls (you can download the source
here). The control allows you to type in text and provides matching items
from a data source (for example a database). This provides the same
functionality as Google
Suggests.
This is an amazingly complex control and you are advised to read the
extensive documentation that is provided to fully explore it's features.
This is a brief introduction to the control to show how easy it is to get it up
and running.
Here are the steps to implement this web control in your own modules. This works with the full version of
Visual Studio 2005 and
also Visual Web Developer Express.
If you haven't already performed this step, you will want to add the DNN web
controls to your Visual Studio toolbox:
- Right-Click on the Toolbox in Visual Studio and select Choose
Items...

- Use the Browse button to browse to DotNetNuke.WebControls.dll (in the
/bin
folder of your DotNetNuke installation) and click the OK button.

- The DotNetNuke web controls will now appear in your toolbox:

- Drag the DNNTextSuggest control from the toolbox and drop it on to your
DotNetNuke Web User Control (See my tutorial
Creating a Super-Simple
DotNetNuke module for information on how to create a DotNetNuke Web User
Control) .
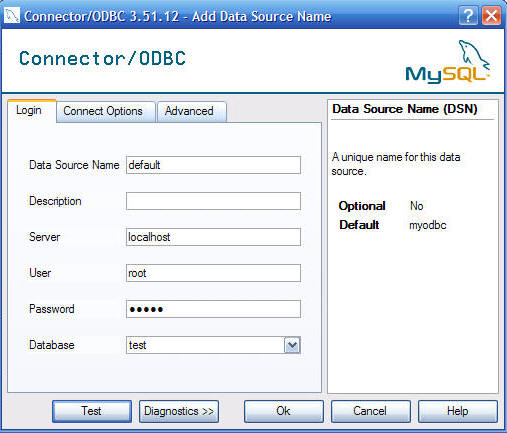
- In the properties for the DNNTextSuggest control, click on the
yellow lazerbolt to show the events for the control
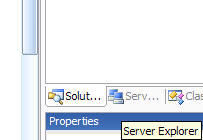
- We see that there are events that we can
wireup a method to. Type "MyDNNTextSuggest_PopulateOnDemand" for the
PopulateOnDemand event and click away from the box.
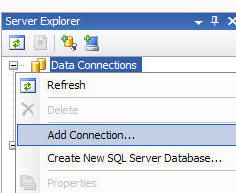
- Visual Studio switches to code view and your method is all ready for
your custom code. Add "PopulateList(e.Nodes, e.Text)"
to the method so it appears like this:
Protected
Sub
MyDNNTextSuggest_PopulateOnDemand(ByVal
source As
Object,
ByVal e
As
DotNetNuke.UI.WebControls.DNNTextSuggestEventArgs)
Handles
MyDNNTextSuggest.PopulateOnDemand
PopulateList(e.Nodes, e.Text)
End
Sub
- Ensure that your Web User Control has this line toward the top:
Inherits Entities.Modules.PortalModuleBase
- Enter this additional code:
Private
Sub PopulateList(ByVal
objNodes As
DNNNodeCollection, ByVal
strText As
String)
Dim o
As DNNNode
Dim dt
As DataTable =
GetData()
'strip out troublesome chars for
Select below
strText = strText.Replace("[",
"").Replace("]",
"").Replace("'",
"''")
dt.CaseSensitive = Me.MyDNNTextSuggest.CaseSensitive
Dim drs()
As DataRow =
dt.Select("name like '"
& strText & "%'")
Dim dr
As DataRow
For
Each dr
In drs
If
Me.MyDNNTextSuggest.MaxSuggestRows
= 0 _
OrElse
objNodes.Count < (Me.MyDNNTextSuggest.MaxSuggestRows
+ 1) Then
o = New
DNNNode(CStr(dr("name")))
o.ID = CStr(dr("id"))
objNodes.Add(o)
End
If
Next
End
Sub
Private Function GetData()
As DataTable
Dim dt
As DataTable =
New DataTable
dt.Columns.Add(New
DataColumn("id",
GetType(String)))
dt.Columns.Add(New
DataColumn("name",
GetType(String)))
AddRow(1, "name1",
dt)
AddRow(2, "name12",
dt)
AddRow(3, "name123",
dt)
AddRow(4, "name1234",
dt)
AddRow(5, "name12345",
dt)
Return dt
End
Function
Private Sub AddRow(ByVal
ID As
Integer,
ByVal Name
As
String,
ByVal dt
As DataTable)
Dim dr
As DataRow = dt.NewRow()
dr("id") = ID
dr("name") =
Name
dt.Rows.Add(dr)
End
Sub
- Save the page and Build the page. When you type "name"
in the text box it will show you matching items.
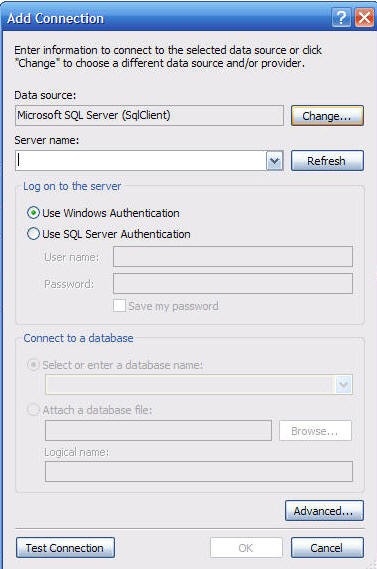
- You can download the code
here.
This example is so simple it really does not do the control justice. However,
you can see that the control is just as easy to use as a Microsoft ASP.NET AJAX
control. In addition, all of the web controls work with ASP.NET 1.1 and
DotNetNuke 3.
Jon Henning continuously develops web controls and provides
excellent
documentation on the DotNetNuke site. I hope to
introduce you to this incredible resource and demonstrate yet another reason why
web development is faster and easier when you use the DotNetNuke Framework.
Also see:
The
ADefWebserver DotNetNuke HELP WebSite